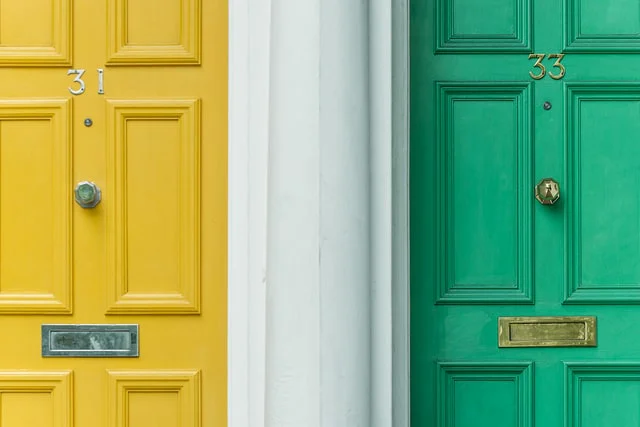
The Monty-Hall-Problem
· 5 minutes reading time Math statistics javascript
Welcome to The Monty-Hall-Show
The Monty-Hall-Show was a popular show back in the day when contestants could go on this game show and might go home with the car of their dreams. The game show host was a very famous guy named Monty Hall. In this problem, which never happened like this in reality, Monty would come on to the show, and he would present three doors. He would give a contestant the chance to pick one of the doors. Behind only one of those doors was the dream car. Behind the other two doors were goats or just something nobody really wants.
It looks like a 1/3 chance of getting a dream car. Suppose the contestant picks door number one and Monty would go over to the two doors she didn’t pick. Since Monty knew exactly where the car was, he would open one of the doors that had a goat behind it. Then Monty would look at the contestant and ask: “Do you still like door number one or do you want to switch”?
There’s only one door left to switch to. Is the contestant going to stay with her choice or is she going to make that leap to the other door? Very often contestants would stand there agonized.
What’s the best strategy?
A remarkable thing about this simple problem is, that it has sparked just endless debate. While the show was running, I don’t recall anybody ever proposed a dedicated strategy. But there is a dedicated strategy one should follow: always switch doors, because it doubles the chances!
There is a 1/3 chance the car is behind the initially picked door. That means there must be a 2/3 chance the car is somewhere else. Since we know that somewhere else cannot be the door that was just opened by Monty, it has to be the other unopened door.
Putting it to the extreme
Unconvinced? There is a way to understand this in a more extreme example. Imagine not having three doors, but 100 doors. Let’s imagine that we’re playing the same kind of game. Monty is going to give the contestant an opportunity to pick a door. There’s only a 1/100 chance the dream car is behind the door that is picked. Compared to the original setting, the chances are now very slim to get the car.
Monty now opens 98 of those other 99 doors. Hint: Monty is not opening one door, he is always opening all remaining doors except one. Behind every door is a goat. He asks the contestant if she wants to switch to that other unopened door. Just because of the sheer number of doors, maybe this is a little clearer.
There is a 99/100 chance the car is behind one of the doors the contestant didn’t pick. With the original setting, some people say that it’s a 50/50 chance if there’re only two unopened doors left, so the contestant can either stay with her initial choice or switch, it doesn’t matter. Would you say the same now with the 100 doors example? Because if you would say so, you’re effectively saying that if you pick one door out of 100 doors, in half of the cases you got a dream car. Ha, you know that is wrong! Even your guts should tell you in the 100 doors example to switch doors. Suddenly it seems like a no-brainer.
Simulation time
Are you still unconvinced? I’ve written a little simulator that can help you with that.
Enter the number of doors, the number of tries, and it will make an experiment and count
the wins for both scenarios for you (keep/switch).
See for yourself. Doors
must be greater than 2 and Tries
must be a positive number.
Wins by strategy
Press calculate ...
Source code
Here is the JavaScript code for the calculation. Alternatively you can look at the page source:
// Play a game
const play = (doors) => {
const carDoor = Math.floor(Math.random() * doors);
const selectedDoor = Math.floor(Math.random() * doors);
const switchDoor = carDoor === selectedDoor
? (carDoor + 1) % doors // Door with goat
: carDoor;
return {
keepWin: selectedDoor === carDoor,
switchWin: switchDoor === carDoor,
};
};
// Simulate some games
const simulate = (doors, tries) => {
const result = [...new Array(tries)]
.map(() => play(doors))
.reduce((agg, curr) => {
if (curr.keepWin) agg['keepWins'] += 1;
if (curr.switchWin) agg['switchWins'] += 1;
return agg;
}, { keepWins: 0, switchWins: 0 });
return {
winPercentKeep: result.keepWins / tries,
winPercentSwitch: result.switchWins / tries,
};
};
// Example output for 1,000 tries with 3 doors
console.log(simulate(3, 1000));
Observations
If you’ve read so far, you might have realised something and asked: “Hey, wait a minute, what are you doing there”? The algorithm for selecting the “switch-to” door directly uses the conclusion. Yes, that’s true: when you try to implement an efficient selection mechanism, you should find that the “switch-to” door has to be the door with the dream car in all the cases where the contestant has initially chosen a door with a goat behind it. That happens in 2/3 of all cases.
Further reading
Read more about the problem on Wikipedia.